Uncontrolled Component in React Js
In every web application, handing form data is very basic requirement and if you want to handle this requirement in react Js, it provides two ways controlled component and uncontrolled component.
According to official document of React Js, it is recommended that in most of cases, controlled component should be implemented for Forms, but in this post, we will discuss about uncontrolled component.
Basic Difference between controlled and uncontrolled component:
a) In a controlled component, form data is handled by a React component.
b) In a uncontrolled component, form data is handled by the DOM itself.
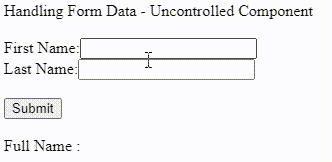 |
Uncontrolled Component in React Js |
Default Values:
With an uncontrolled component, if you want to specify the initial value, you can specify a defaultValue attribute instead of value.
Sample Code:
A) Class Component
a) App.js
import React, { Component } from 'react';
class App extends Component {
constructor(props) {
super(props);
this.handleSubmit = this.handleSubmit.bind(this);
this.inputFirstName = React.createRef();
this.inputLastName = React.createRef();
this.state = {fullName: ''}
}
handleSubmit(event) {
const name = this.inputFirstName.current.value + ' ' +
this.inputLastName.current.value;
this.setState({fullName: name});
event.preventDefault();
}
render() {
return (
<form onSubmit={this.handleSubmit}>
<label>
Handling Form Data - Uncontrolled Component
</label> <br /><br />
<label>
First Name:
<input defaultValue="vikas" type="text" ref={this.inputFirstName} />
</label> <br />
<label>
Last Name:
<input defaultValue="gore" type="text" ref={this.inputLastName} />
</label><br /><br />
<input type="submit" value="Submit" /> <br /> <br />
<label>
Full Name :
{this.state.fullName}
</label>
</form>
);
}
}
export default App;
B) Functional Component
a) App.js
import React, { useRef, useState } from 'react';
export default function App() {
const inputFirstName = useRef('');
const inputLastName = useRef('');
const [fullName, setFullName] = useState('');
function handleSubmit(event) {
const name =
inputFirstName.current.value + ' ' + inputLastName.current.value;
setFullName(name);
event.preventDefault();
}
return (
<form onSubmit={handleSubmit}>
<label>Handling Form Data - Uncontrolled Component</label> <br />
<br />
<label>
First Name:
<input defaultValue="vikas" type="text" ref={inputFirstName} />
</label>{' '}
<br />
<label>
Last Name:
<input defaultValue="gore" type="text" ref={inputLastName} />
</label>
<br />
<br />
<input type="submit" value="Submit" /> <br /> <br />
<label>Full Name :{fullName}</label>
</form>
);
}
Happy Learning!! Happy Coding!!
Comments